출처 : 애플 공식문서 https://developer.apple.com/swift/
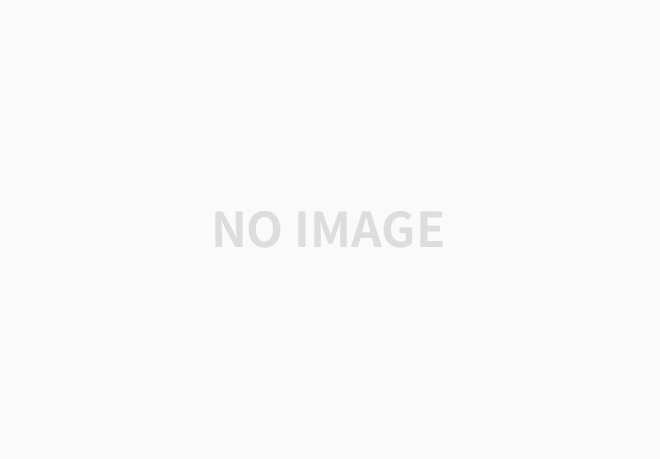
The powerful programming language that is also easy to learn.
Swift is a powerful and intuitive programming language for iOS, iPadOS, macOS, tvOS, and watchOS. Writing Swift code is interactive and fun, the syntax is concise yet expressive, and Swift includes modern features developers love. Swift code is safe by design, yet also produces software that runs lightning-fast.
손쉽게 학습할 수 있는 강력한 프로그래밍 언어.
Swift는 macOS, iOS, watchOS, tvOS를 위한 강력하고 직관적인 프로그래밍 언어입니다. Swift 코드 작성은 대화식으로 재미있고, 구문은 간결하면서도 표현력이 풍부하며, Swift에는 개발자들이 좋아하는 첨단 기능이 포함되어 있습니다. Swift 코드는 안전하게 설계되었으며 빛의 속도로 빠르게 실행되는 소프트웨어도 제작할 수 있습니다.
Modern 최신 기능
Swift is the result of the latest research on programming languages, combined with decades of experience building Apple platforms. Named parameters are expressed in a clean syntax that makes APIs in Swift even easier to read and maintain. Even better, you don’t even need to type semi-colons. Inferred types make code cleaner and less prone to mistakes, while modules eliminate headers and provide namespaces. To best support international languages and emoji, Strings are Unicode-correct and use a UTF-8 based encoding to optimize performance for a wide-variety of use cases. Memory is managed automatically using tight, deterministic reference counting, keeping memory usage to a minimum without the overhead of garbage collection.
Swift는 프로그래밍 언어에 대한 최신 연구 결과이며 수십 년에 걸친 Apple 플랫폼 구축 경험이 결합되어 있습니다. 명명된 매개변수는 Swift로 작성된 API를 더 쉽게 읽고 유지 관리할 수 있게 해주는 명확한 구문으로 표현됩니다. 세미콜론도 입력할 필요가 없습니다. 추론된 유형은 코드를 더 명확하게 만들고 실수를 덜 하도록 도와주며, 모듈은 헤더를 없애고 이름 공간을 제공합니다. 문자열은 다국어 및 이모티콘 지원을 위해 유니코드 표준을 따르며 다양한 사례에 사용할 수 있도록 성능을 최적화하기 위해 UTF-8 기반의 인코딩을 사용합니다. 엄격하고 확정적인 참조 계수를 사용하고, 가비지 컬렉션 관련 오버헤드 없이 최소한의 메모리 사용량을 유지하여 메모리를 자동으로 관리합니다.
struct Player {
var name: String
var highScore: Int = 0
var history: [Int] = []
init(_ name: String) {
self.name = name
}
}
var player = Player("Tomas")
Declare new types with modern, straightforward syntax. Provide default values for instance properties and define custom initializers.
복잡하지 않은 최신 구문으로 새로운 유형을 선언합니다. 인스턴스 속성을 위한 기본 값을 제공하고 맞춤형 이니셜라이저를 정의합니다.
extension Player {
mutating func updateScore(_ newScore: Int) {
history.append(newScore)
if highScore < newScore {
print("\(newScore)! A new high score for \(name)! 🎉")
highScore = newScore
}
}
}
player.updateScore(50)
// Prints "50! A new high score for Tomas! 🎉"
// player.highScore == 50
Add functionality to existing types using extensions, and cut down on boilerplate with custom string interpolations.
확장 프로그램을 사용하여 기존 유형에 기능성을 더하고, 맞춤형 문자열 보간으로 상용구를 줄입니다.
extension Player: Codable, Equatable {}
import Foundation
let encoder = JSONEncoder()
try encoder.encode(player)
print(player)
// Prints "Tomas, games played: 1, high score: 50
Quickly extend your custom types to take advantage of powerful language features, such as automatic JSON encoding and decoding.
자동화된 JSON 인코딩 및 디코딩과 같은 강력한 언어 기능을 활용하도록 맞춤형 유형을 빠르게 확장합니다.
let players = getPlayers()
// Sort players, with best high scores first
let ranked = players.sorted(by: { player1, player2 in
player1.highScore > player2.highScore
})
// Create an array with only the players’ names
let rankedNames = ranked.map { $0.name }
// ["Erin", "Rosana", "Tomas"]
Perform powerful custom transformations using streamlined closures.
간결한 클로저로 강력한 맞춤형 변환을 수행합니다.
These forward-thinking concepts result in a language that is fun and easy to use.
Swift has many other features to make your code more expressive:
이러한 미래 지향적인 개념은 재미있고 사용하기 쉬운 언어를 만들어 냅니다.
Swift는 다음과 같이 코드의 표현력을 높이기 위한 다른 많은 기능을 제공합니다.
- Generics that are powerful and simple to use
- Protocol extensions that make writing generic code even easier
- First class functions and a lightweight closure syntax
- Fast and concise iteration over a range or collection
- Tuples and multiple return values
- Structs that support methods, extensions, and protocols
- Enums can have payloads and support pattern matching
- Functional programming patterns, e.g., map and filter
- Native error handling using try / catch / throw
- 강력하고 사용이 간편한 제네릭
- 프로토콜 확장 프로그램으로 보다 쉬워진 제네릭 코드 작성
- 1급 함수 및 가벼운 클로저 구문
- 범위 또는 컬렉션에서의 빠르고 간결한 반복
- 튜플 및 멀티플 반환 값
- 메소드, 확장 프로그램 및 프로토콜을 지원하는 구조
- 페이로드를 포함할 수 있으며 패턴 일치를 지원하는 enum
- 함수형 프로그래밍 패턴(예:지도 및 필터)
- try/catch/throw를 사용한 기본 오류 처리
Designed for Safety
안전 중심 설계
Swift eliminates entire classes of unsafe code. Variables are always initialized before use, arrays and integers are checked for overflow, memory is automatically managed, and enforcement of exclusive access to memory guards against many programming mistakes. Syntax is tuned to make it easy to define your intent — for example, simple three-character keywords define a variable ( var ) or constant ( let ). And Swift heavily leverages value types, especially for commonly used types like Arrays and Dictionaries. This means that when you make a copy of something with that type, you know it won’t be modified elsewhere.
Another safety feature is that by default Swift objects can never be nil. In fact, the Swift compiler will stop you from trying to make or use a nil object with a compile-time error. This makes writing code much cleaner and safer, and prevents a huge category of runtime crashes in your apps. However, there are cases where nil is valid and appropriate. For these situations Swift has an innovative feature known as optionals. An optional may contain nil, but Swift syntax forces you to safely deal with it using the ? syntax to indicate to the compiler you understand the behavior and will handle it safely.
Swift는 불안전한 코드의 전체 클래스를 제거합니다. 변수는 사용 전에 항상 초기화되고, 배열 및 정수에 대한 오버플로우 검사가 수행되며, 메모리는 자동으로 관리됩니다. 또한 메모리에 대한 독점적인 접근을 통해 많은 프로그래밍 실수가 발생하지 않도록 보호합니다. 구문은 사용자의 의도를 쉽게 정의할 수 있도록 조정됩니다. 예를 들어 3자로 된 간단한 키워드는 변수(var) 또는 상수(let)를 정의합니다. 뿐만 아니라 Swift는 특히 배열 및 딕셔너리와 같이 일반적으로 사용되는 값 유형을 최대한 활용합니다. 이는 해당 유형으로 사본을 만들 경우 다른 곳에서 수정할 수 없다는 것을 의미합니다.
또 다른 안전 기능은 기본적으로 Swift 객체가 결코 nil이 될 수 없게 하는 것입니다. 실제로 Swift 컴파일러는 컴파일 시 오류가 있는 nil 객체를 만들거나 사용할 수 없도록 합니다. 이렇게 하면 코드를 훨씬 깔끔하고 안전하게 작성할 수 있으며 앱에서 거대한 카테고리의 런타임 충돌을 방지할 수 있습니다. 하지만 nil이 유효하고 적절한 경우도 있습니다. 이러한 경우를 위해 Swift는 선택 사항(Optional)이라는 혁신적인 기능을 제공합니다. 선택 사항에는 nil이 포함될 수 있지만 Swift 구문은 ? 구문을 사용하여 nil을 안전하게 처리하도록 함으로써 컴파일러에 동작을 이해하고 안전하게 처리될 것임을 표시합니다.
extension Collection where Element == Player {
// Returns the highest score of all the players,
// or `nil` if the collection is empty.
func highestScoringPlayer() -> Player? {
return self.max(by: { $0.highScore < $1.highScore })
}
}
Use optionals when you might have an instance to return from a function, or you might not.
함수에서 반환할 인스턴스가 있거나 없을 때 선택 사항을 이용할 수 있습니다.
if let bestPlayer = players.highestScoringPlayer() {
recordHolder = """
The record holder is \(bestPlayer.name),\
with a high score of \(bestPlayer.highScore)!
"""
} else {
recordHolder = "No games have been played yet.")
}
print(recordHolder)
// The record holder is Erin, with a high score of 271!
let highestScore = players.highestScoringPlayer()?.highScore ?? 0
// highestScore == 271
Features such as optional binding, optional chaining, and nil coalescing let you work safely and efficiently with optional values.
바인딩 선택 사항, 체이닝 선택 사항 등의 기능과 nil 통합을 사용하면 선택 사항 값으로 안전하고 효율적으로 작업할 수 있습니다.
Fast and Powerful
빠르고 강력한 성능
From its earliest conception, Swift was built to be fast. Using the incredibly high-performance LLVM compiler technology, Swift code is transformed into optimized native code that gets the most out of modern hardware. The syntax and standard library have also been tuned to make the most obvious way to write your code also perform the best whether it runs in the watch on your wrist or across a cluster of servers.
Swift is a successor to both the C and Objective-C languages. It includes low-level primitives such as types, flow control, and operators. It also provides object-oriented features such as classes, protocols, and generics, giving Cocoa and Cocoa Touch developers the performance and power they demand.
Swift는 최초 개념 설정 시점부터 빠르게 동작하도록 만들어졌습니다. Swift 코드는 뛰어난 고성능 LLVM 컴파일러 기술을 사용하여 최신 하드웨어를 최대한 활용할 수 있도록 최적화된 기본 코드로 변환됩니다. 또한 구문 및 표준 라이브러리는 손목에 착용한 시계에서든 서버 클러스터 전반에서든, 코드를 작성하는 가장 확실한 방법으로, 최고의 성능을 발휘하도록 조정되었습니다.
Swift는 C 및 Objective-C 언어의 후속 언어로 유형, 흐름 제어, 연산자와 같은 하위 수준 프리미티브를 포함합니다. 또한 클래스, 프로토콜, 제네릭과 같은 객체 지향 기능을 제공하므로 Cocoa 및 Cocoa Touch 개발자에게 필요한 성능과 파워를 제공합니다.
Great First Language
탁월한 제1언어
Swift can open doors to the world of coding. In fact, it was designed to be anyone’s first programming language, whether you’re still in school or exploring new career paths. For educators, Apple created free curriculum to teach Swift both in and out of the classroom. First-time coders can download Swift Playgrounds—an app for iPad that makes getting started with Swift code interactive and fun.
Swift는 코딩의 세계로 들어가기 위한 관문입니다. 실제로 Swift는 아직 공부 중인 학생이나 새로운 진로를 탐색하는 사람, 누구나 사용할 수 있는 최초의 프로그래밍 언어로 개발되었습니다. Apple은 교육자들을 위해 강의실 안팎에서 Swift를 가르치기 위한 무료 커리큘럼을 개설했습니다. 코딩 입문자들은 Swift 코드를 사용하여 대화식으로 즐겁게 시작할 수 있는 iPad용 앱인 Swift Playgrounds를 다운로드할 수 있습니다.
Aspiring app developers can access free courses to learn to build their first apps in Xcode. And Apple Stores around the world host Today at Apple Coding & Apps sessions where you can get hands-on experience with Swift code.
앱 개발자 지망생들은 무료 과정을 통해 Xcode로 첫 번째 앱을 빌드하는 방법을 학습할 수 있습니다.뿐만 아니라 전 세계의 Apple Store에서는 Swift 코드를 직접 경험해 볼 수 있는 Today at Apple 코딩 및 앱 세션을 주최합니다.
Learn more about Swift education resources from Apple
Apple의 Swift 교육 리소스 더 알아보기(영문)
Source and Binary Compatibility
소스 및 바이너리 호환성
With Swift 5, you don’t have to modify any of your Swift 4 code to use the new version of the compiler. Instead you can start using the new compiler and migrate at your own pace, taking advantage of new Swift 5 features, one module at a time. And Swift 5 now introduces binary compatibility for apps. That means you no longer need to include Swift libraries in apps that target current and future OS releases, because the Swift libraries will be included in every OS release going forward. Your apps will leverage the latest version of the library in the OS, and your code will continue to run without recompiling. This not only makes developing your app simpler, it also reduces the size of your app and its launch time.
Swift 5를 사용하면 새로운 버전의 컴파일러를 사용하기 위해 Swift 4 코드를 수정할 필요가 없습니다. 대신 새로운 컴파일러를 사용하여 한 번에 한 모듈씩 새로운 Swift 5 기능을 활용하여 자신만의 속도로 마이그레이션할 수 있습니다. 또한 Swift 5는 앱에 대한 바이너리 호환성을 도입합니다. 즉, Swift 라이브러리가 향후 릴리즈될 모든 OS에 포함되므로 현재 및 향후 OS 릴리즈를 대상으로 하는 앱에 Swift 라이브러리를 포함하지 않아도 됩니다. 앱은 OS에서 최신 라이브러리 버전을 활용하게 되며 코드 역시 다시 컴파일할 필요 없이 계속 실행 가능합니다. 따라서 앱을 보다 간단하게 개발하면서 앱의 크기도 줄고 실행 시간도 단축됩니다.
Open Source
오픈 소스
Swift is developed in the open at Swift.org, with source code, a bug tracker, forums, and regular development builds available for everyone. This broad community of developers, both inside Apple as well as hundreds of outside contributors, work together to make Swift even more amazing. There is an even broader range of blogs, podcasts, conferences and meetups where developers in the community share their experiences of how to realize Swift’s great potential.
Swift는 Swift.org에서 오픈 소스로 개발되었으며 모든 사람이 이용할 수 있는 소스 코드, 버그 추적기, 포럼 및 정기적인 개발 빌드가 포함되어 있습니다. Apple뿐만 아니라 수백 명의 외부 개발자가 참여하는 이 광범위한 개발자 커뮤니티에서 Swift를 더욱 멋진 언어로 만들기 위해 협력하고 있습니다. 커뮤니티는 개발자들이 Swift의 놀라운 잠재력을 실현한 경험을 공유하는 다양한 블로그, 팟캐스트, 컨퍼런스 및 모임으로 구성됩니다.
Cross Platform 크로스 플랫폼
Swift already supports all Apple platforms and Linux, with community members actively working to port to even more platforms. With SourceKit-LSP, the community is also working to integrate Swift support into a wide-variety of developer tools. We’re excited to see more ways in which Swift makes software safer and faster, while also making programming more fun.
Swift는 Linux를 비롯한 모든 Apple 플랫폼을 지원하며, 더 많은 플랫폼을 지원하기 위해 커뮤니티 회원들이 활발하게 활동하고 있습니다. 커뮤니티에서는 SourceKit-LSP를 사용하여 Swift 지원을 다양한 개발자 도구로 통합하는 작업도 수행합니다. Apple은 Swift로 소프트웨어를 더 빠르고 안전하게 만드는 동시에 프로그래밍을 더 재미있게 만드는 다양한 방법을 모색할 수 있어 기쁘게 생각합니다.
Swift for Server 서버를 위한 Swift
While Swift powers many new apps on Apple platforms, it’s also being used for a new class of modern server applications. Swift is perfect for use in server apps that need runtime safety, compiled performance and a small memory footprint. To steer the direction of Swift for developing and deploying server applications, the community formed the Swift Server work group. The first product of this effort was SwiftNIO, a cross-platform asynchronous event-driven network application framework for high performance protocol servers and clients. It serves as the foundation for building additional server-oriented tools and technologies, including logging, metrics and database drivers which are all in active development.
Swift는 Apple 플랫폼에서 수많은 신규 앱을 강력하게 지원하는 동시에 새로운 클래스의 최신 서버 응용 프로그램에도 사용됩니다. Swift는 런타임 안정성, 컴파일된 성능 및 소규모 메모리 공간이 필요한 서버 앱에 사용하기에 적합합니다. 커뮤니티에서는 Swift를 서버 응용 프로그램 개발 및 배포용으로 활용할 수 있도록 Swift 서버 작업 그룹을 구성했습니다. 이러한 노력의 첫 번째 성과로 고성능 프로토콜 서버 및 클라이언트를 위한 크로스 플랫폼 비동기식 이벤트 중심 네트워크 응용 프로그램 프레임워크인 SwiftNIO가 탄생했습니다. SwiftNIO는 로깅, 메트릭 및 데이터베이스 드라이버를 비롯한 추가 서버 지향 도구 및 기술 구축을 위한 기반으로, 현재 모두 활발하게 개발이 진행 중입니다.
To learn more about the open source Swift community and the Swift Server work group, visit Swift.org
오픈 소스 Swift 커뮤니티 및 Swift 서버 작업 그룹에 대해 자세히 알아보려면 Swift.org(영문)를 방문하십시오.
Playgrounds and Read-Eval-Print-Loop (REPL)
Much like Swift Playgrounds for iPad, playgrounds in Xcode make writing Swift code incredibly simple and fun. Type a line of code and the result appears immediately. You can then Quick Look the result from the side of your code, or pin that result directly below. The result view can display graphics, lists of results, or graphs of a value over time. You can open the Timeline Assistant to watch a complex view evolve and animate, great for experimenting with new UI code, or to play an animated SpriteKit scene as you code it. When you’ve perfected your code in the playground, simply move that code into your project. Swift is also interactive when you use it in Terminal or within Xcode’s LLDB debugging console. Use Swift syntax to evaluate and interact with your running app, or write new code to see how it works in a script-like environment.
iPad용 Swift Playgrounds와 마찬가지로 Xcode의 Playground를 사용하여 매우 간단하고 재미있게 Swift 코드를 작성할 수 있으며, 한 줄의 코드를 입력하면 결과가 즉시 나타납니다. 작성 후에는 코드 옆에서 Quick Look(훑어보기) 기능으로 결과를 확인하거나 해당 결과를 바로 아래에 고정할 수 있습니다. 결과 보기에 그래픽, 결과 목록 또는 시간 경과에 따른 값의 그래프를 표시할 수 있습니다. Timeline Assistant를 열면 복잡한 뷰가 변하고 움직이는 것을 볼 수 있어 새로운 UI 코드를 실험하는 데 유용하며, 코드를 작성하면서 움직이는 SpriteKit 장면을 재생할 수 있습니다. 플레이그라운드에서 코드를 완벽하게 만들었다면 코드를 프로젝트로 이동하기만 하면 됩니다. Swift는 터미널 또는 Xcode의 LLDB 디버깅 콘솔에서도 상호 작용이 가능합니다. Swift 구문을 사용하여 실행 중인 앱을 평가하고 이러한 앱과 상호 작용하거나, 새로운 코드를 작성하여 스크립트와 유사한 환경에서 어떻게 작동하는지 확인할 수 있습니다.
Package Manager
Swift Package Manager is a single cross-platform tool for building, running, testing and packaging your Swift libraries and executables. Swift packages are the best way to distribute libraries and source code to the Swift community. Configuration of packages is written in Swift itself, making it easy to configure targets, declare products and manage package dependencies. New to Swift 5, the swift run command now includes the ability to import libraries in a REPL without needing to build an executable. Swift Package Manager itself is actually built with Swift and included in the Swift open source project as a package.
Swift Package Manager는 Swift 라이브러리 및 실행 파일을 구축, 실행, 테스트 및 패키징하기 위한 단일 크로스 플랫폼 도구입니다. Swift 패키지는 Swift 커뮤니티에 라이브러리 및 소스 코드를 배포하는 가장 좋은 방법입니다. 패키지 구성은 Swift로 직접 작성되어 손쉽게 대상을 구성하고, 제품을 결정하며, 패키지 종속성을 관리할 수 있습니다. Swift 5에 새롭게 추가된 Swift 실행 명령에는 실행 파일을 빌드하지 않고도 REPL에서 라이브러리를 가져올 수 있는 기능이 포함됩니다. Swift Package Manager는 실제로 Swift로 빌드되며 Swift 오픈 소스 프로젝트에 패키지 형태로 포함됩니다.
Objective-C Interoperability 상호 운용성
You can create an entirely new application with Swift today, or begin using Swift code to implement new features and functionality in your app. Swift code co-exists along side your existing Objective-C files in the same project, with full access to your Objective-C API, making it easy to adopt.
지금 바로 Swift를 사용하여 전혀 새로운 응용 프로그램을 만들거나 Swift 코드 사용을 시작하여 앱에 새로운 기능을 구현할 수 있습니다. 동일한 프로젝트에서 기존 Objective-C 파일과 함께 Swift 코드가 공존하며, Objective-C API에 대한 완전한 접근 권한이 제공되므로 적용이 용이합니다.
'모바일앱 > Swift' 카테고리의 다른 글
Scope (0) | 2021.09.28 |
---|---|
튜플(Tuple) (0) | 2021.09.28 |
코멘트 (0) | 2021.09.28 |
컬렉션 타입(Array, Dictionary, Set) (0) | 2021.09.27 |
Any, AnyObject, nil (0) | 2021.09.27 |
기본 데이터 타입 (0) | 2021.09.26 |
상수와 변수 (0) | 2021.09.26 |
명명법 / 콘솔로그 / 문자열 보간법 (0) | 2021.09.26 |